javascript,function aStar(startNode, endNode, graph) {, let openSet = [startNode];, let cameFrom = new Map();, , let gScore = new Map();, gScore.set(startNode, 0);, , let fScore = new Map();, fScore.set(startNode, heuristic(startNode, endNode));, , while (openSet.length > 0) {, let current = openSet.reduce((a, b) => fScore.get(a)< fScore.get(b) ? a : b);, , if (current === endNode) {, return reconstructPath(cameFrom, current);, }, , openSet = openSet.filter(node => node !== current);, , for (let neighbor of graph.getNeighbors(current)) {, let tentativeGScore = gScore.get(current) + graph.getCost(current, neighbor);, , if (!gScore.has(neighbor) || tentativeGScore< gScore.get(neighbor)) {, cameFrom.set(neighbor, current);, gScore.set(neighbor, tentativeGScore);, fScore.set(neighbor, tentativeGScore + heuristic(neighbor, endNode));, , if (!openSet.includes(neighbor)) {, openSet.push(neighbor);, }, }, }, }, , return null; // No path found,},,function heuristic(nodeA, nodeB) {, // Example heuristic function (Manhattan distance), return Math.abs(nodeA.x nodeB.x) + Math.abs(nodeA.y nodeB.y);,},,function reconstructPath(cameFrom, current) {, let totalPath = [current];, while (cameFrom.has(current)) {, current = cameFrom.get(current);, totalPath.unshift(current);, }, return totalPath;,},
``,,这个代码实现了A*算法的基本逻辑,包括开放集管理、代价计算以及路径重建。你可以根据具体需求调整启发函数和其他细节。在当今的软件开发领域,算法是实现高效和优化性能的关键,B星寻路算法(B-star Algorithm)是一种广泛使用的路径搜索算法,尤其在游戏开发、机器人导航和地图服务中,本文将深入探讨B星寻路算法及其JavaScript实现。
B星寻路算法
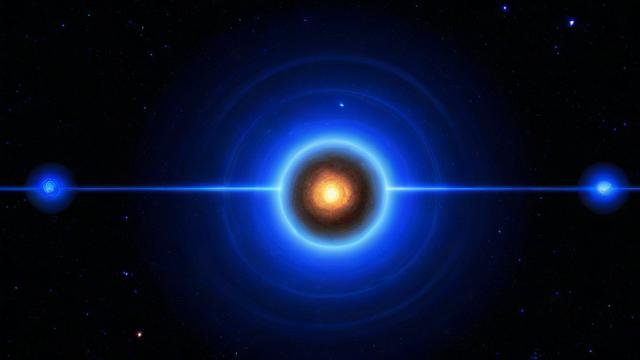
B星寻路算法是一种启发式搜索算法,用于在图中找到从起点到终点的最短路径,它结合了Dijkstra算法的最优性和A*算法的启发性,通过评估函数来选择路径,该评估函数包括已走过的距离和估计的剩余距离。
核心概念
1、启发函数(Heuristic Function):通常使用曼哈顿距离或欧几里得距离来估计当前节点到目标节点的距离。
2、开放列表(Open List):存储待处理的节点,按启发值排序。
3、关闭列表(Closed List):存储已处理的节点,避免重复处理。
4、g(n):从起点到当前节点的实际成本。
5、h(n):从当前节点到终点的估计成本。
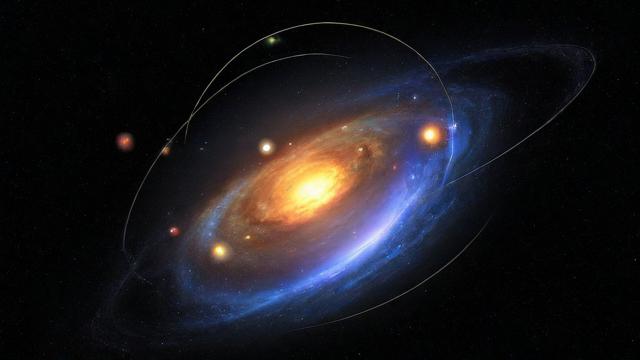
6、f(n) = g(n) + h(n):评估函数,用于决定节点的优先级。
JavaScript实现
以下是一个简单的JavaScript实现示例,展示了如何使用B星寻路算法在一个二维网格上找到路径。
class Node { constructor(x, y, parent = null) { this.x = x; this.y = y; this.parent = parent; this.g = 0; this.h = 0; this.f = 0; } } function heuristic(node, end) { // 使用曼哈顿距离作为启发函数 return Math.abs(node.x end.x) + Math.abs(node.y end.y); } function bStarSearch(start, end, grid) { let openList = []; let closedList = new Set(); start.h = heuristic(start, end); start.f = start.h; openList.push(start); while (openList.length > 0) { openList.sort((a, b) => a.f b.f); let current = openList.shift(); if (current.x === end.x && current.y === end.y) { return reconstructPath(current); } closedList.add(current); const neighbors = getNeighbors(current, grid); for (let neighbor of neighbors) { if (closedList.has(neighbor)) continue; let tentativeG = current.g + 1; if (!openList.some(node => node.x === neighbor.x && node.y === neighbor.y && node.g <= tentativeG)) { neighbor.parent = current; neighbor.g = tentativeG; neighbor.h = heuristic(neighbor, end); neighbor.f = neighbor.g + neighbor.h; openList.push(neighbor); } } } return null; // No path found } function getNeighbors(node, grid) { let neighbors = []; const directions = [[-1, 0], [1, 0], [0, -1], [0, 1]]; for (let [dx, dy] of directions) { let nx = node.x + dx; let ny = node.y + dy; if (nx >= 0 && ny >= 0 && nx < grid.length && ny < grid[0].length && grid[nx][ny] !== 1) { // Assuming '1' is an obstacle neighbors.push(new Node(nx, ny)); } } return neighbors; } function reconstructPath(node) { let path = []; while (node) { path.push({ x: node.x, y: node.y }); node = node.parent; } return path.reverse(); }
应用案例
假设我们有一个5x5的网格,其中一些单元格被标记为障碍物(值为1),其余为可通行区域(值为0),起点在左上角(0,0),终点在右下角(4,4),我们可以使用上述代码来计算从起点到终点的最短路径。
let grid = [ [0, 0, 0, 0, 0], [0, 1, 1, 1, 0], [0, 0, 0, 0, 0], [0, 1, 1, 1, 0], [0, 0, 0, 0, 0] ]; let start = new Node(0, 0); let end = new Node(4, 4); let path = bStarSearch(start, end, grid); console.log(path); // 输出路径坐标数组
相关问答FAQs
Q1: B星寻路算法的时间复杂度是多少?
A1: B星寻路算法的时间复杂度取决于开放列表的大小,最坏情况下可以达到O(b^d),其中b是分支因子(每个节点的平均子节点数),d是搜索空间的维度,由于启发函数的使用,实际运行时间通常远低于最坏情况。
Q2: 如果启发函数低估了实际距离会怎样?
A2: 如果启发函数低估了实际距离,B星寻路算法仍然可以找到最短路径,但效率可能会降低,因为它可能探索更多的节点,如果启发函数总是低估实际距离,算法保证能找到最优解,但可能不是以最快的方式。
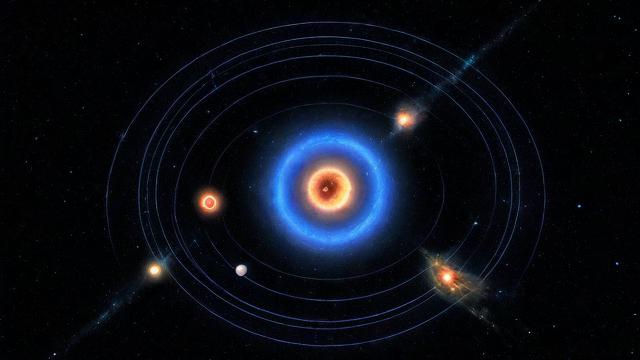
到此,以上就是小编对于“b星寻路算法js”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。