pthread_join
函数来等待一个线程结束。这个函数会阻塞调用它的线程,直到指定的线程终止。在Linux操作系统中,线程是轻量级的进程,它们共享相同的地址空间和资源,有时需要等待一个线程执行完成再继续执行主线程中的其他任务,本文将探讨如何在Linux中实现等待线程结束的方法。
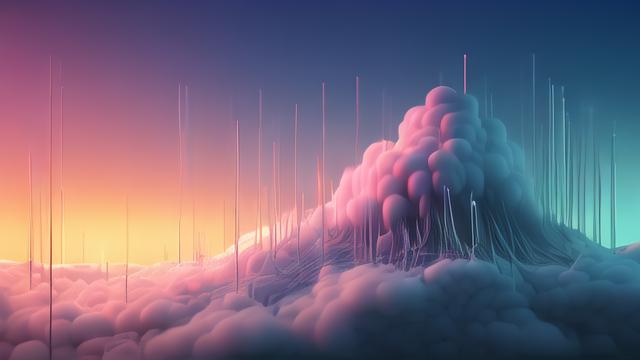
方法一:使用pthread_join
函数
pthread_join
是一个用于等待指定线程终止的函数,它接受两个参数:线程标识符(即线程ID)和一个指向线程返回值的指针,当被等待的线程结束时,主线程将继续执行。
#include <pthread.h> #include <stdio.h> #include <stdlib.h> void* thread_function(void* arg) { printf("Thread is running... "); sleep(2); // 模拟耗时操作 printf("Thread has finished execution. "); return NULL; } int main() { pthread_t thread; int result; // 创建一个新线程 if (pthread_create(&thread, NULL, thread_function, NULL) != 0) { perror("Failed to create thread"); exit(EXIT_FAILURE); } // 等待线程结束 if (pthread_join(thread, NULL) != 0) { perror("Failed to join thread"); exit(EXIT_FAILURE); } printf("Main thread continues after waiting for the child thread to finish. "); return 0; }
在这个例子中,我们创建了一个新的线程来执行thread_function
函数,并使用pthread_join
等待这个线程结束,一旦子线程完成其任务,主线程将打印一条消息并继续执行。
方法二:使用条件变量和互斥锁
另一种方法是使用条件变量和互斥锁来同步线程,条件变量允许一个或多个线程阻塞,直到另一个线程发出信号,互斥锁则用于保护共享数据结构,防止竞争条件的发生。
#include <pthread.h> #include <stdio.h> #include <stdlib.h> pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER; pthread_cond_t cond = PTHREAD_COND_INITIALIZER; int ready = 0; void* thread_function(void* arg) { printf("Thread is running... "); sleep(2); // 模拟耗时操作 // 锁定互斥锁并设置ready标志 pthread_mutex_lock(&mutex); ready = 1; pthread_cond_signal(&cond); // 发送信号以唤醒等待的线程 pthread_mutex_unlock(&mutex); printf("Thread has finished execution. "); return NULL; } int main() { pthread_t thread; // 创建一个新线程 if (pthread_create(&thread, NULL, thread_function, NULL) != 0) { perror("Failed to create thread"); exit(EXIT_FAILURE); } // 锁定互斥锁并等待ready标志被设置 pthread_mutex_lock(&mutex); while (!ready) { pthread_cond_wait(&cond, &mutex); // 等待条件变量的信号 } pthread_mutex_unlock(&mutex); printf("Main thread continues after waiting for the child thread to finish. "); return 0; }
在这个例子中,我们使用了条件变量和互斥锁来实现线程间的同步,子线程在完成任务后会设置ready
标志并通过条件变量发送信号给主线程,主线程则会在收到信号后继续执行。
FAQs
Q1: 为什么需要等待线程结束?
A1: 在某些情况下,主线程可能需要等待子线程完成其任务后再继续执行,如果子线程负责计算结果或处理数据,那么主线程可能需要等待这些结果才能进行下一步操作,等待线程结束还可以确保所有资源都被正确释放,避免内存泄漏等问题。
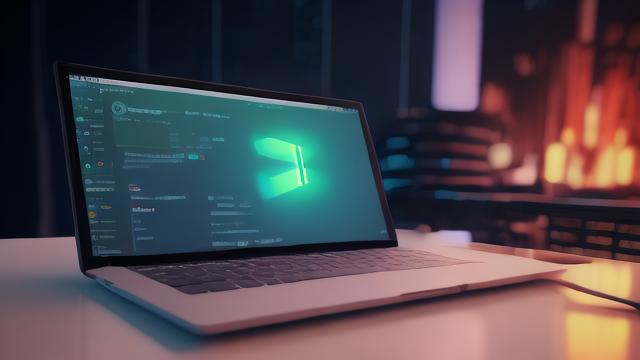
Q2:pthread_join
和条件变量/互斥锁有什么区别?
A2:pthread_join
是一个简单而直接的方法来等待线程结束,它适用于那些只需要等待单个线程完成的场景,而条件变量和互斥锁提供了更灵活的同步机制,可以用于复杂的多线程应用程序中,通过条件变量和互斥锁,可以实现更细粒度的控制,例如让多个线程等待同一个条件或者在不同的条件下唤醒不同的线程。
以上就是关于“linux等待线程结束”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!