在数据库编程中,fetchall
是一个常用的方法,用于从查询结果集中检索所有记录,本文将详细介绍fetchall
的使用方法、注意事项以及相关示例。
一、`fetchall` 的基本概念

fetchall
是大多数数据库连接库提供的一个方法,用于执行 SQL 查询后获取所有匹配的记录,它通常返回一个包含多个元组或字典(取决于使用的数据库库)的列表,每个元组或字典代表一行数据。
二、常见数据库库中的fetchall
1、MySQL (使用mysql-connector-python
)
import mysql.connector # 建立数据库连接 conn = mysql.connector.connect( host="localhost", user="yourusername", password="yourpassword", database="yourdatabase" ) cursor = conn.cursor() # 执行查询 cursor.execute("SELECT * FROM your_table") # 使用 fetchall 获取所有记录 results = cursor.fetchall() for row in results: print(row) # 关闭连接 cursor.close() conn.close()
2、PostgreSQL (使用psycopg2
)
import psycopg2 # 建立数据库连接 conn = psycopg2.connect( dbname="yourdatabase", user="yourusername", password="yourpassword", host="localhost" ) cursor = conn.cursor() # 执行查询 cursor.execute("SELECT * FROM your_table") # 使用 fetchall 获取所有记录 results = cursor.fetchall() for row in results: print(row) # 关闭连接 cursor.close() conn.close()
3、SQLite (使用内置的sqlite3
模块)
import sqlite3 # 建立数据库连接 conn = sqlite3.connect('your_database.db') cursor = conn.cursor() # 执行查询 cursor.execute("SELECT * FROM your_table") # 使用 fetchall 获取所有记录 results = cursor.fetchall() for row in results: print(row) # 关闭连接 cursor.close() conn.close()
三、使用表格展示fetchall
的结果
假设我们有一个名为employees
的表,结构如下:
CREATE TABLE employees ( id INT PRIMARY KEY, name VARCHAR(50), department VARCHAR(50), salary DECIMAL(10, 2) );
插入一些示例数据:
INSERT INTO employees (id, name, department, salary) VALUES (1, 'Alice', 'HR', 60000), (2, 'Bob', 'Engineering', 70000), (3, 'Charlie', 'Marketing', 55000);
使用fetchall
获取所有员工记录并以表格形式展示:
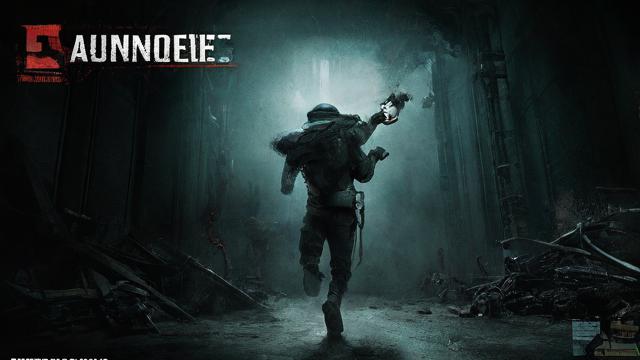
import sqlite3 建立数据库连接 conn = sqlite3.connect(':memory:') cursor = conn.cursor() 创建表并插入数据 cursor.execute(""" CREATE TABLE employees ( id INT PRIMARY KEY, name VARCHAR(50), department VARCHAR(50), salary DECIMAL(10, 2) ) """) cursor.executemany("INSERT INTO employees (id, name, department, salary) VALUES (?, ?, ?, ?)", [ (1, 'Alice', 'HR', 60000), (2, 'Bob', 'Engineering', 70000), (3, 'Charlie', 'Marketing', 55000) ]) conn.commit() 执行查询并使用 fetchall 获取所有记录 cursor.execute("SELECT * FROM employees") results = cursor.fetchall() 打印表头 print(f"{'ID':<5} {'Name':<10} {'Department':<15} {'Salary':<10}") print("="*40) 打印每一行数据 for row in results: print(f"{row[0]:<5} {row[1]:<10} {row[2]:<15} {row[3]:<10.2f}") 关闭连接 cursor.close() conn.close()
输出结果如下:
ID Name Department Salary 1 Alice HR 60000.00 2 Bob Engineering 70000.00 3 Charlie Marketing 55000.00
四、常见问题及解答 (FAQs)
Q1:fetchall
和fetchone
有什么区别?
A1:fetchall
和fetchone
都是用于从查询结果集中检索数据的方法。fetchall
返回所有匹配的记录,而fetchone
只返回第一条匹配的记录,如果结果集中没有记录,fetchall
返回一个空列表,而fetchone
返回None
。
Q2: 如果查询结果非常多,使用fetchall
会导致什么问题?
A2: 如果查询结果非常多,使用fetchall
可能会导致内存不足的问题,因为fetchall
会将所有结果一次性加载到内存中,在这种情况下,建议使用fetchmany
分批获取数据,或者逐行处理数据以避免内存占用过高,可以使用fetchone
在循环中逐行读取数据。
五、小编有话说
fetchall
是一个非常有用的方法,可以快速获取查询结果中的所有记录,在实际开发中,我们需要根据具体情况选择合适的方法来处理查询结果,对于大量数据,可以考虑使用分页或批量处理的方式,以确保应用程序的性能和稳定性,希望本文对你有所帮助,如果你有任何疑问或建议,欢迎在评论区留言讨论!